TOC
Understanding Numpy Transpose
1.Transpose is to switch the row and column indices of the matrix A;
x = np.arange(8).reshape((4,2))
print(x)
print(x.T)
# output:
# [[0 1] # x
# [2 3]
# [4 5]
# [6 7]]
# [[0 2 4 6] # x.T
# [1 3 5 7]]
x = np.arange(9).reshape((3,3))
print(x)
print(x.T)
print(x.shape, x.T.shape)
# output:
# [[0 1 2]
# [3 4 5]
# [6 7 8]]
# [[0 3 6]
# [1 4 7]
# [2 5 8]]
x = np.arange(8).reshape((2,4))
print(x)
print(x.T)
# output:
# [[0 1 2 3]
# [4 5 6 7]]
# [[0 4]
# [1 5]
# [2 6]
# [3 7]]
If the array has only one dimension, the transpose of the array will not change;
x = np.arange(8).reshape((8))
print(x) # output: [0 1 2 3 4 5 6 7]
print(x.T) # output: [0 1 2 3 4 5 6 7]
Flips the shape
2.Transpose a array will switch the shape number along the central axis; for example:
import numpy as np
x = np.arange(8).reshape((8))
print(x.shape, x.T.shape) # output: (8,) (8,)
x = np.arange(8).reshape((2,4))
print(x.shape, x.T.shape) # output: (2, 4) (4, 2)
x = np.arange(24).reshape((2,3,4))
print(x.shape, x.T.shape) # output: (2, 3, 4) (4, 3, 2)
x = np.arange(120).reshape((2,3,4,5))
print(x.shape, x.T.shape) # output: (2, 3, 4, 5) (5, 4, 3, 2)
REFERENCE: 1.Transpose
「点个赞」
点个赞
使用微信扫描二维码完成支付
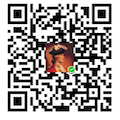