TOC
How does numpy add two arrays with different shapes?
Numpy has a add
method which add two numpy array.
Arithmetic operation +
does the same thing as Numpy.add
;
1.Add a same shapes array
Let’s see a example.
import numpy as np
list1 = np.array([1, 2, 3]);
list2= np.array([10, 20, 30]);
print("list1:",list1,"list2:",list2);
# Print: list1: [1 2 3] list2: [10 20 30]
added_list = list1 + list2;
print("added_list.shape:",added_list.shape,"\nadded_list:",added_list);
# Print:
# added_list.shape: (3,)
# added_list: [11 22 33]
added_list = np.add(list1, list2);
print("added_list.shape:",added_list.shape,"\nadded_list:",added_list);
# Print:
# added_list.shape: (3,)
# added_list: [11 22 33]
2.Add a different shape array
But what happen if two array have different shapes?
Following example show this case,
import numpy as np
list1 = np.array([[1, 1, 1]]);
list2 = np.array([10]);
added_list = list1 + list2; # Print: [[11 11 11]]
The smaller array is “broadcast” across the larger array so that they have compatible shapes
- The Broadcasting Rule:
In order to broadcast, the size of the trailing axes for both arrays in an operation must either be the same size or one of them must be one.
Numpy will add two arrays element-by-element, dimension-by-dimension.
If arrays didn’t have the same dimension when numpy add
, numpy will stretch the smaller dimension to match the larger one conceptually.
Note: the smaller dimension must be one dimension.
Examples of the output shape :
list1.shape is (2,1,5)
list2.shape is (1,2,1)
The output shape is (2,2,5)
#----------
list1.shape is (1, 9, 4)
list2.shape is (1444, 1, 4)
The output shape is (1444, 9, 4)
The examples of adding with different shape:
import numpy as np
list1 = np.array([[[1, 1, 1, 1,],
[2, 2, 2, 2,],
[3, 3, 3, 3,]]]);
list2 = np.array([[[ 10, 10, 10, 10]],
[[ 100, 100, 100, 100]],
[[ 1000,1000,1000,1000]],
]);
print("list1.shape:",list1.shape,"\nlist2.shape:",list2.shape);
# Print:
# list1.shape: (1, 3, 4)
# list2.shape: (3, 1, 4)
added_list = list1 + list2
print("added_list.shape:",added_list.shape,"\nadded_list:",added_list);
# Print:
# added_list.shape: (3, 3, 4)
# added_list: [[[ 11 11 11 11]
# [ 12 12 12 12]
# [ 13 13 13 13]]
# [[ 101 101 101 101]
# [ 102 102 102 102]
# [ 103 103 103 103]]
# [[1001 1001 1001 1001]
# [1002 1002 1002 1002]
# [1003 1003 1003 1003]]]
For this example, the first dimension, which is 1, will be extended to 3 to match the first one of list2 ( the shape of list2 is (3,1,4) );
# list1 expand to this shape
list1 = np.array([[[1, 1, 1, 1,],
[2, 2, 2, 2,],
[3, 3, 3, 3,]],
[[1, 1, 1, 1,],
[2, 2, 2, 2,],
[3, 3, 3, 3,]],
[[1, 1, 1, 1,],
[2, 2, 2, 2,],
[3, 3, 3, 3,]]]);
And the second dimension of list2 is 1, which will be extended to 3 to match the second dimension of list1, so list2 look like this:
list2 = np.array([[[ 10, 10, 10, 10],
[ 10, 10, 10, 10],
[ 10, 10, 10, 10]],
[[ 100, 100, 100, 100],
[ 100, 100, 100, 100],
[ 100, 100, 100, 100]],
[[ 1000,1000,1000,1000],
[ 1000,1000,1000,1000],
[ 1000,1000,1000,1000]],
]);
Python version: 3.7.9
Numpy version: 1.18.5
REFERENCE: 1.theory.Broadcasting
「点个赞」
点个赞
使用微信扫描二维码完成支付
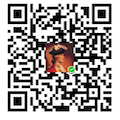