TOC
Comparison Of Programing Language
Here are the release dates of the following programming languages:
-
C: C was first released on March 8, 1972.
-
Objective-C: Objective-C was first released on 1984.
-
C++: C++ was first released in 1985.
-
Python: Python was first released on February 20, 1991.
-
Java: Java was first released on May 23, 1995.
-
PHP: PHP was first released on June 8, 1995.
-
Dart: Dart was first announced on October 10, 2011, and its first stable version was released on November 14, 2013.
-
Swift: Swift was first announced and released on June 2, 2014.
These programming languages have since evolved with newer versions and updates being released over time to keep them up to date with the latest trends and technology advancements. Let me know if there’s anything else I can help you with.
Primitive data type
-
C: int/float/double/char/bool; modifiers: signed/unsigned/short/long
-
C++: int/float/double/char/bool; modifiers: signed/unsigned/short/long
-
Objective-C: short/int/long/float/double/char/BOOL/void; modifiers: signed/unsigned
-
Python: int/float/complex/str/bool/bytes;
-
Java: byte/short/int/long/float/double/boolean/char
-
PHP: Integer/Float/Boolean/String/Array/Object/NULL
-
Dart: int/double/num/String/bool/List/Set/Map/Runes
-
Swift: Int/Float/Double/Bool/String/Character/UInt
Commonality 1: C languages all have int, char, float, and double, as well as short and long. The difference is that in Objective-C, short and long are treated as basic types, not just modifiers.
共同点:都有int/(float or double)/bool
区别:
- C: string is a library; signed/unsigned/short/long可以修饰int,决定长度;
- C++: string is a library; signed/unsigned/short/long可以修饰int,决定长度;
- Python: 没有double
- Java: byte/short/long 是单独的int类型;string是对象
- PHP: 没有double;
- Dart: double=float
- Swift: 数据类型首字母都大写
String data type
- C: char array
char greetings[] = "Hello World!";
printf("%c", greetings[0]);
- C++:
- Python:
- Java:
- PHP:
- Dart:
- Swift:
Thread
- C: C itself is not single-threaded; it can support multithreading by using threading libraries such as POSIX Threads (pthreads), Windows Threads, or other platform-specific libraries
// gcc -o pthread_example pthread_example.c -lpthread
// ./pthread_example
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#define NUM_THREADS 3 // Define the number of threads you want to create
// Define the function that each thread will execute
void *print_message(void *thread_id) {
long tid = (long) thread_id;
printf("Hello from thread %ld\n", tid);
pthread_exit(NULL); // End the thread
}
int main() {
pthread_t threads[NUM_THREADS]; // Array to hold thread identifiers
int rc; // Return code from pthread functions
long t;
// Create NUM_THREADS threads
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, print_message, (void *) t);
if (rc) {
// If there is an error, print the error code and exit
printf("Error: pthread_create failed with code %d\n", rc);
exit(-1);
}
}
// Wait for all threads to complete
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
printf("All threads completed.\n");
return 0;
}
- C++: C++ itself does not inherently manage concurrency or multithreading, C++ provides a standard library (, , , <condition_variable>, etc.) that offers tools for managing threads, synchronization, and parallelism.
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(threadFunction); // Create a new thread
t.join(); // Wait for the thread to complete
return 0;
}
Threads can be managed using methods such as join() (to wait for a thread to finish) or detach() (to allow a thread to run independently).
- Python: Python is primarily single-threaded due to its implementation’s use of the Global Interpreter Lock (GIL). Python’s threading module provides high-level support for creating and managing threads. You can create and manage multiple threads, but due to the GIL, threads primarily achieve concurrency (not parallelism) in Python.
from concurrent.futures import ProcessPoolExecutor
import random
import time
def square_number(number):
"""Function that squares a given number"""
time.sleep(1) # Simulating a time-consuming operation
return number ** 2
def main():
# List of numbers to square
numbers = [random.randint(1, 10) for _ in range(10)]
# Create a ProcessPoolExecutor with 4 worker processes
with ProcessPoolExecutor(max_workers=4) as executor:
# Submit tasks to the executor and collect Future objects
future_to_number = {executor.submit(square_number, num): num for num in numbers}
# Retrieve results as they complete
for future in future_to_number:
number = future_to_number[future]
try:
# Get the result of the future
result = future.result()
print(f"The square of {number} is {result}")
except Exception as exc:
print(f"Generated an exception: {exc}")
if __name__ == '__main__':
main()
- Java: Java is a programming language that natively supports multithreaded programming, providing high-level abstractions and tools for creating and managing threads, synchronization, and parallelism. Java’s java.lang.Thread class provides support for creating and managing threads.
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import java.util.stream.Collectors;
public class ParallelismExample {
public static void main(String[] args) {
// Create a list of integers
List<Integer> numbers = new ArrayList<>();
Random random = new Random();
// Populate the list with random numbers
for (int i = 0; i < 10000; i++) {
numbers.add(random.nextInt(100));
}
// Use parallelStream() to create a parallel stream from the list
List<Integer> filteredNumbers = numbers.parallelStream()
// Filter the list to include only even numbers
.filter(number -> number % 2 == 0)
// Sort the filtered numbers in descending order
.sorted((a, b) -> b - a)
// Collect the results into a list
.collect(Collectors.toList());
// Print the filtered and sorted list
System.out.println("Filtered and sorted numbers (only even numbers):");
System.out.println(filteredNumbers);
}
}
-
Dart: Dart is designed to be a single-threaded language with asynchronous capabilities.
-
Swift
create a thread.
import Foundation
// Define a function to be executed in a separate thread
func performExpensiveTask() {
// Simulate an expensive task
sleep(5)
print("Expensive task completed on thread: \(Thread.current)")
}
// Create a new thread and start the expensive task
let newThread = Thread {
performExpensiveTask()
}
// Start the new thread
newThread.start()
// Print a message on the main thread
print("Main thread continues running...")
Using DispatchQueue.
import Foundation
// Define a function to simulate a time-consuming task
func performTask(id: Int) {
print("Task \(id) started")
// Simulate some work using a sleep
sleep(UInt32(id))
print("Task \(id) completed")
}
// Define the main function
func main() {
// Create a concurrent queue using GCD
let concurrentQueue = DispatchQueue(label: "com.example.concurrentQueue", attributes: .concurrent)
// Dispatch tasks to the concurrent queue
for i in 1...5 {
concurrentQueue.async {
performTask(id: i)
}
}
// Add a small delay to allow tasks to complete before the program exits
// In a real-world scenario, you may want to use DispatchGroup or other synchronization primitives
Thread.sleep(forTimeInterval: 6)
}
// Call the main function
main()
「点个赞」
点个赞
使用微信扫描二维码完成支付
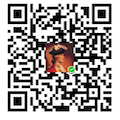